学习目标
在此项目中,您将:
- 构建一组简单的Lightning Web组件。
- 从单个记录,然后从记录列表中检索并显示数据。
- 将功能封装到子组件中。
- 使用事件进行跨组件通信。
介绍
在这个项目中,您将通过创建一个让公园管理员跟踪熊的应用程序来尝试Lightning Web Components的基本概念。
Lightning Web Components是用于构建Lightning组件的新编程模型。它使用最新的Web标准,并且可以与原始的Aura编程模型互操作。
在此项目中,您为虚拟国家公园Ursus Park工作。您创建的应用程序允许护林员跟踪在公园中游荡的熊。
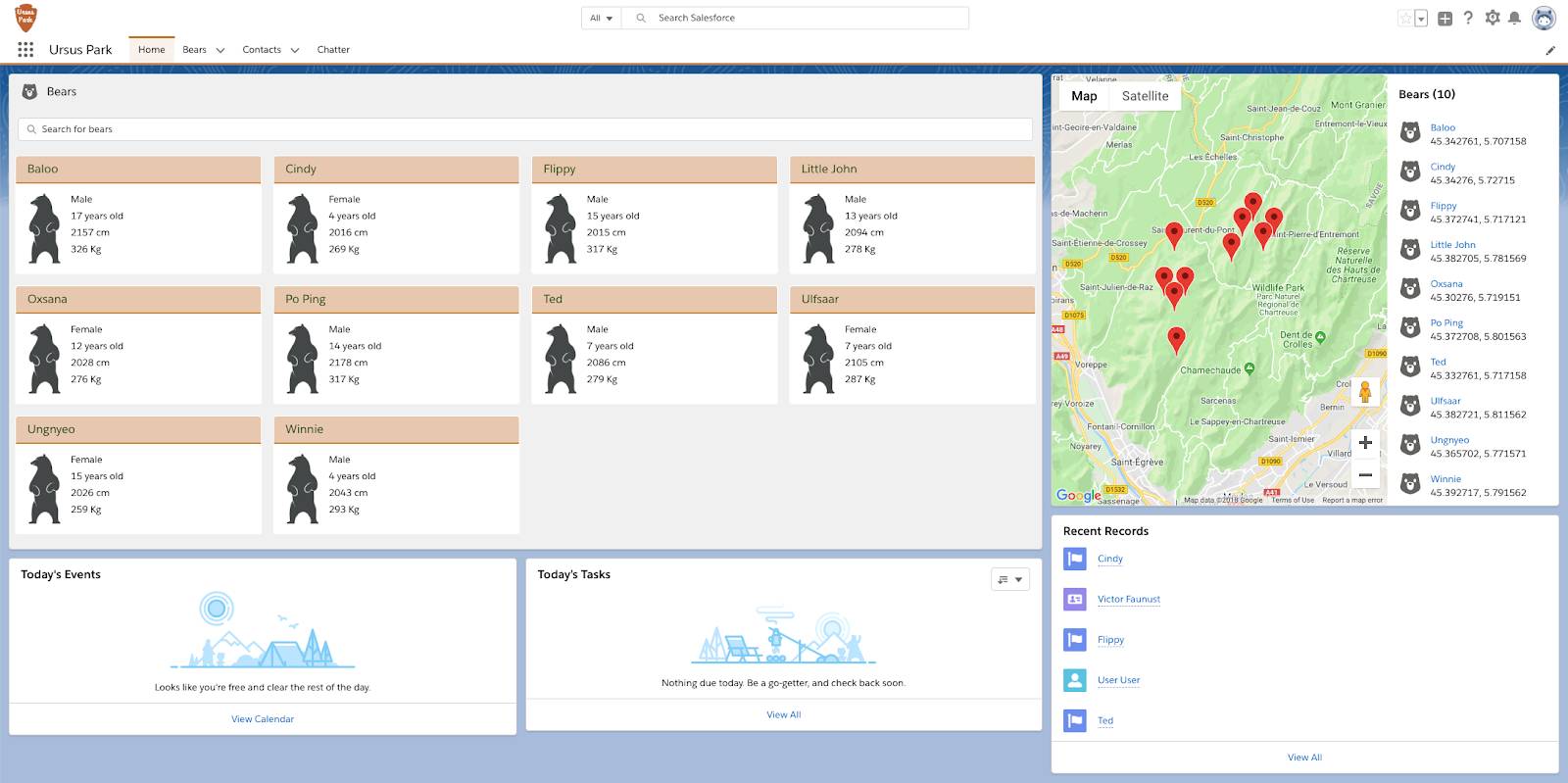
在你开始之前
在进行此项目之前,请确保完成“快速入门:闪电Web组件”中的这些步骤 。如果您尚未完成快速入门中的步骤,则将无法完成该项目。
确保已安装VS Code和Salesforce CLI。
设置您的Trailhead游乐场
- 要创建新的Trailhead Playground,请点击此步骤末尾的下拉菜单,然后选择Create a Trailhead Playground。
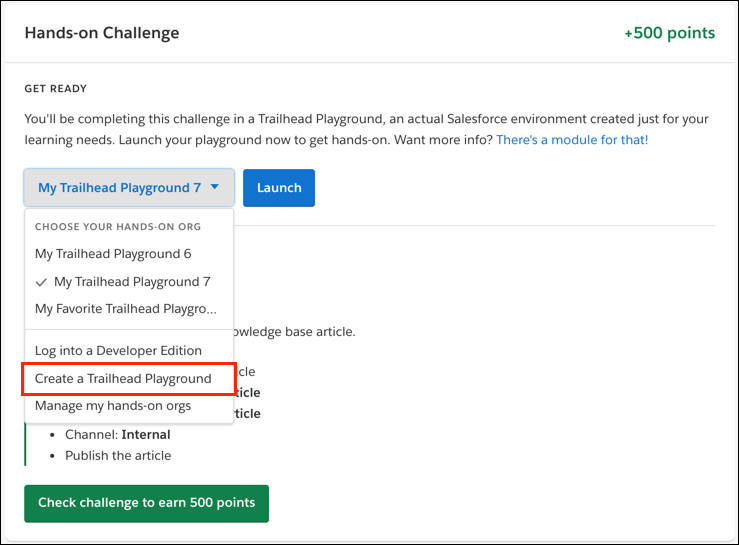
- 拥有Trailhead游乐场后,单击“启动”。
如果您在组织中看到一个标签为“获取登录凭据”的标签,那就太好了!请按照以下步骤操作。
如果不是,请打开App Launcher(
),找到并选择Playground Starter,然后按照以下步骤操作。如果您没有看到Playground Starter应用程序,请在Trailhead帮助中签出 查找Trailhead Playground的用户名和密码。
- 单击获取您的登录凭据选项卡,并记下您的用户名。
- 单击重置我的密码。这会将电子邮件发送到与您的用户名关联的地址。
- 单击电子邮件中的链接。
- 输入新密码,确认,然后单击更改密码。
设置Ursus Park应用
- 打开命令提示符,例如Windows上的cmd或MacOS上的Terminal。
- 克隆Ursus Park应用程序git存储库。
该存储库包含Ursus Park应用程序,具有一组字段,记录和页面布局的Bear对象以及用于检索熊记录和样本熊记录的Apex代码。该项目基础帮助我们专注于Lightning Web Component开发。请注意,VS Code集成了Git支持,您也可以直接从开放源代码站点(https://git-scm.com/)安装它。
- 导航到新的build-apps-with-lwc目录。
- 使用Salesforce CLI授权Trailhead Playground,使用
bear-tracking
别名保存它,并将当前用户设置为默认用户:
- 当带有Salesforce登录页面的浏览器窗口打开时,输入您的Trailhead Playground凭据。
- 将应用程序代码部署到组织中。
- 将Ursus Park用户权限集分配给当前用户。
- 导入样本数据。
- 在浏览器中打开组织。
- 在应用启动器(
)中,找到并选择Ursus Park。这将打开闪电应用程序。
- 单击Bears选项卡,并确保已填充一些示例数据。
使用静态HTML创建Hello World Lightning Web组件
让我们创建第一个Lightning Web组件:一个简单的hello world组件。
- 打开VS Code。
- 通过单击文件>打开 文件夹并导航到该
build-apps-with-lwc
文件夹 ,添加刚从GitHub克隆的项目文件夹。
- 在边栏中,展开
force-app/main/default
文件夹。
- 在以下位置创建一个
lwc
文件夹force-app/main/default
- 右键单击该
lwc
文件夹,然后单击SFDX:创建Lightning Web组件并命名该组件helloWebComponent
。
或者,您可以通过sfdx force:lightning:component:create --type lwc -n helloWebComponent -d force-app/main/default/lwc
在命令提示符下运行来获得相同的结果。
- 将内容替换为
helloWebComponent.html
以下标记。
该标记使用静态文本定义了 卡片基础组件。
- 编辑
helloWebComponent.js-meta.xml
文件,并替换<isExposed>false</isExposed>
为这些行。
这些额外的行允许您将组件添加到Lightning App Builder中的任何类型的Lightning页面。
- 将更新的代码部署到组织。在VS Code中,右键单击默认文件夹,然后单击SFDX:将源部署到组织。
将Hello World组件添加到页面
现在我们已经实现了组件,让我们将其添加到页面中以对其进行查看。
- 在浏览器中打开组织:
- 在应用启动器(
)中,找到并选择Ursus Park。这将打开应用程序主页。
- 单击齿轮图标(
),然后选择“编辑页面”。
- 在“自定义组件”下,找到您的helloWebComponent组件并将其拖动到右侧列的顶部。
- 点击保存。
- 由于这是我们第一次修改标准主页,因此我们需要激活更新的页面,以便我们的用户可以看到我们所做的事情。点击激活。
- 单击应用程序默认选项卡。
- 单击分配给应用程序。
- 检查Ursus公园。
- 单击下一步,然后单击保存。
- 单击上一步
返回到主页。
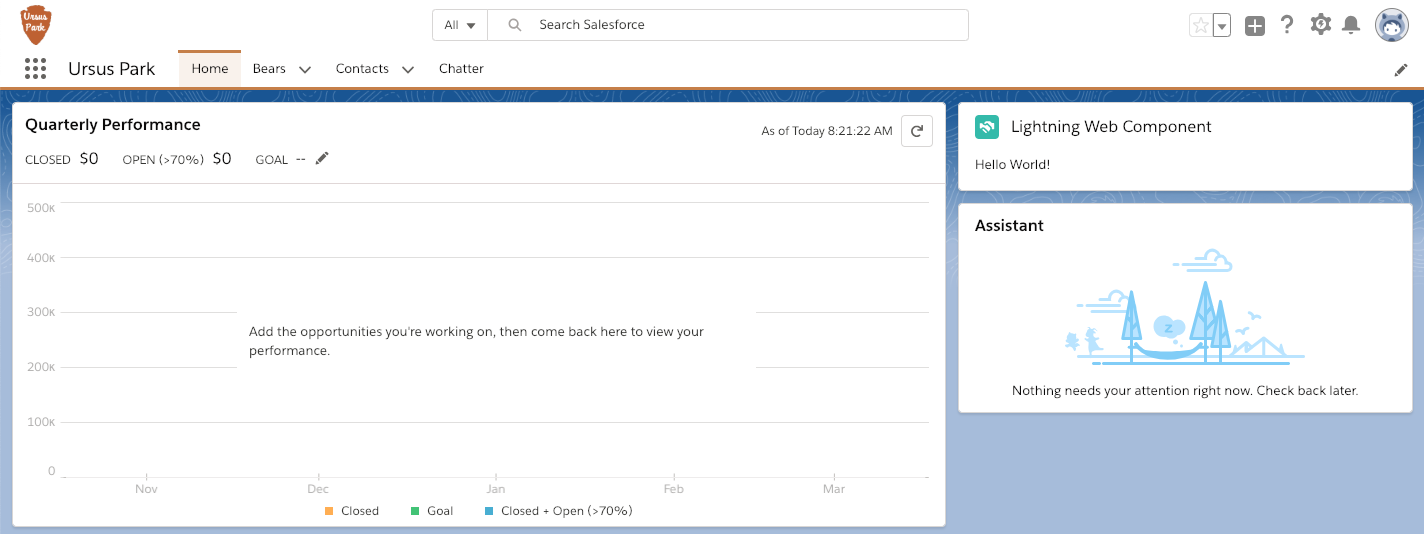
恭喜你!您刚刚创建了第一个Lightning Web组件并将其添加到Lightning Experience中的页面。这个第一个版本并没有太大的作用,所以让我们将其转变为更具动态性的东西。
使用数据绑定
让我们向组件中添加一些数据。我们将使用单向数据绑定。我们首先显示只读数据,然后使其可编辑。
- 在“ VS代码”中,单击
helloWebComponent.html
以对其进行编辑。替换<h2>Hello World!</h2>
为<h2>Hello {greeting}!</h2>
。这将为greeting
我们在下一步中定义的属性添加绑定。
- 编辑
helloWebComponent.js
其内容并替换为以下内容。
这将声明并初始化一个greeting
属性。此属性是反应性的。换句话说,只要greeting
更改值,组件的HTML模板就会自动刷新。
- 将更新的代码部署到组织。右键单击默认文件夹,然后单击SFDX:将源部署到组织。
- 在组织中,刷新Ursus Park主页,并注意您的组件已更改(它现在显示“ Hello Trailblazer!”而不是“ Hello World!”)。
做得好!您已经实现了数据绑定:greeting
读取并显示了该属性,但是用户暂时无法修改该属性。
让我们更进一步,并通过添加输入字段使数据可编辑。
- 在VS Code中,编辑
helloWebComponent.html
并在下面添加以下行<h2>Hello {greeting}!</h2>
:
这段代码添加了一个用SLDS样式化的文本输入字段。输入使用greeting
属性初始化。每当输入更改时,它将调用handleGreetingChange
我们在下一步中定义的JavaScript函数。
- 编辑
helloWebComponent.js
并在下方添加以下行greeting = 'Trailblazer';
。
这定义了一个函数,该函数从事件(来自输入字段的输入更改事件)中捕获值并将其分配给greeting
属性。
- 将更新的代码部署到组织。右键单击默认文件夹,然后单击SFDX:将源部署到组织。
- 在组织中,刷新主页,并注意在编辑文本字段时如何立即更新组件。
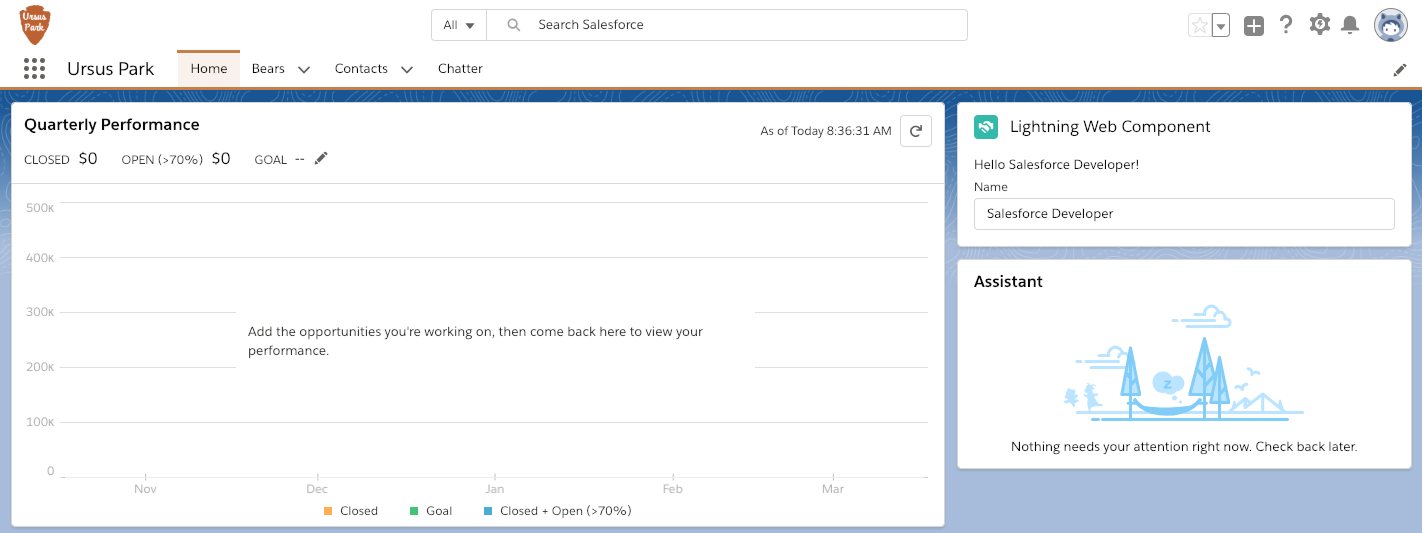
您已建立数据绑定,并使数据可编辑。greeting
每当您在输入字段中更改其值时,该属性就会显示并自动刷新。
显示属性只是一个开始,但是如果需要在呈现之前转换其值怎么办?Lightning Web Components使您可以使用表达式。
使用表达式
让我们进入一些更高级的主题,并使用表达式来显示动态值。我们将添加一个表达式,该表达式以大写字母返回您的名字的问候消息。
- 在VS Code中,编辑
helloWebComponent.html
并替换title="Lightning Web Component"
为title={capitalizedGreeting}
。
- 替换
<h2>Hello {greeting}!</h2>
为<p>Today is {currentDate}</p>
。
- 编辑
helloWebComponent.js
并在handleGreetingChange
功能块上方添加以下几行。
这定义了一个currentDate
类属性和一个capitalizedGreeting
getter函数。这些getter函数称为表达式。它们用于显示值,就像属性一样,但是表达式值可以基于函数中编写的某些逻辑来计算。与属性不同,表达式不是被动的:每次重新渲染组件时,表达式都会自动重新评估。无论其值是否更改,这都是正确的。当用户在组件的输入中键入内容时,handleGreetingChange
事件处理程序函数将更新greeting
属性的值。由于greeting
是反应性的,因此会触发重新渲染,从而迫使表达式重新评估。请注意,我们声明了currentDate
class属性,用于保存和显示当前日期,而不使用表达式。我们也可以使用just编写表达式,return new Date().toDateString();
但是使用属性的好处是不必Date
为每个重新渲染创建新对象。
- 将更新的代码部署到组织。右键单击默认文件夹,然后单击SFDX:将源部署到组织。
- 在组织中,刷新“主页”并注意表达式的显示方式。
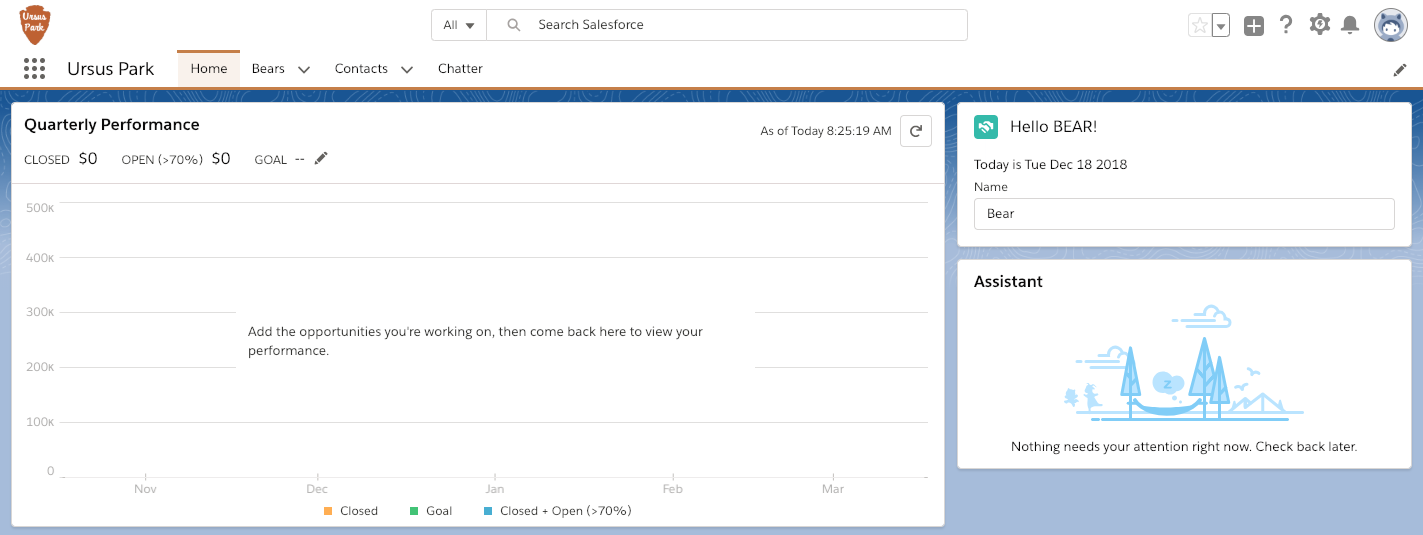
如您所见,表达式使我们能够使组件模板保持逻辑清晰。实际上,使用表达式是在显示属性值之前对其进行转换的唯一方法。
这就是步骤。您已经建立了带有基本组件,数据绑定和表达式的基本hello world组件。
现在我们已经涵盖了基础知识,让我们继续进行一些更令人兴奋的事情。